Overview
Having a light/dark theme toggle can be a powerful accessibility feature in your website. In this article I am going to show you how you can toggle light/dark theme easily using JavaScript to manipulate color variables.
Understanding Variables
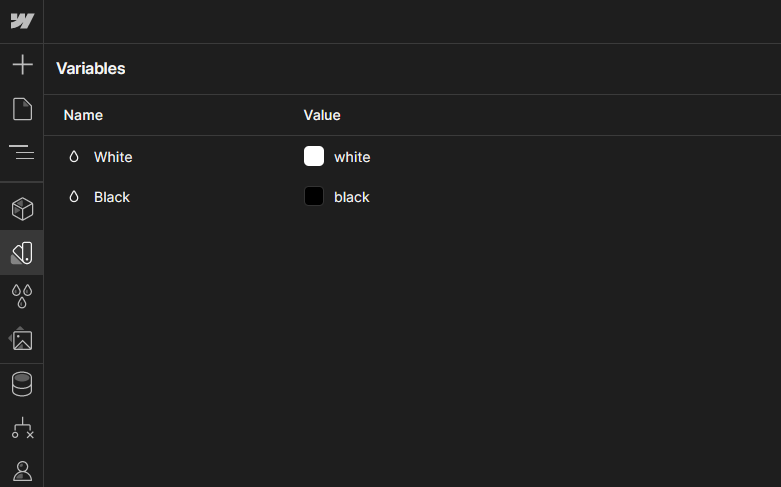
Imagine we have only 2 variables e.g. black and white. as shown in the image. how is this translated in CSS? Variables are attached to your root. In HTML, the root element is <html>. It is the outermost element of an HTML document and contains all the content on the web page.
Now, if we are using variables across the website for all coloring then it's easy to apply dark-mode with a simple trick. We can just manipulate these variables to the dark-mode version. e.g. lightmode: (black text on white bg) -> darkmode: (white text on black bg). So, if we swapped the hex value for black and white variables we will end up having a darkmode.
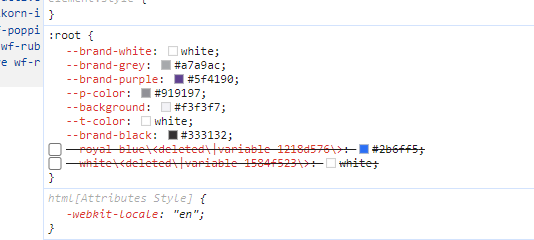
How to manipulate the variables?
You can easily change the hex color by defining a css class and attaching this class to the <body> to override the :root values.
Let's see an example ^^
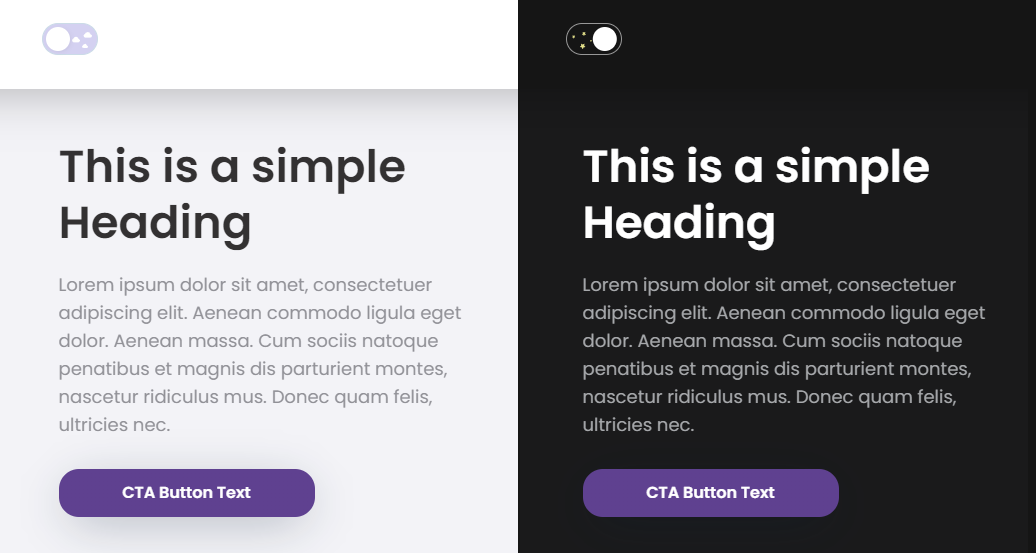
Here I am using the colors shown in image #2 for Lightmode and the colors in the css class dark-mode from the code above for Darkmode .. All we have to do is add the ".dark-mode" class to the body when the theme toggle button is clicked. Easy Peasy huh? Let's see how can we do it using JavaScript.
The provided code manages the switching between dark and light themes on a webpage. It uses a toggle button and stores the user's preference as a cookie to maintain the chosen theme across page reloads. The 'setTheme' function applies the selected theme, and the 'window.onload' event ensures that the stored theme preference is respected when the page initially loads. The 'click' event listener on the toggle button triggers the theme switch.
Note: You can also store the user prereference as a variable in the local storage if you don't want to use cookies.
Happy designing ^^